Host SignalR 2.0 in WPF Application
Microsoft has released new versions of ASP.NET, Visual Studio, SignalR etc. The real-time web might be focused on their SignalR tech since SignalR supports Web Sockets and other compatible techs.
Our team will have a new web project which needs us host SignalR in a WPF application. We searched online long time but most of SignalR Self-hosting samples only indicated how to host SignalR in a Console application.
Microsoft ASP.NET website has provided a great SignalR Self-hosting sample by Patrick Fletcher, I’ve tried to convert his console sample to a WPF sample. There were some troubles to move Patrick’s code to my WPF project, but finally I could let my sample work.
Here I give my steps:
(Note: Let me steal Patrick’s code because I just wanted to convert his sample to a WPF sample, but I try to contribute my different parts )
1: Use Visual Studio 2013, create a WPF application:
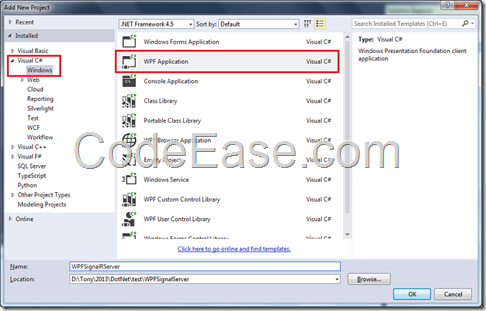
2: Right Click the WPF project, and select Manage NuGet Package…
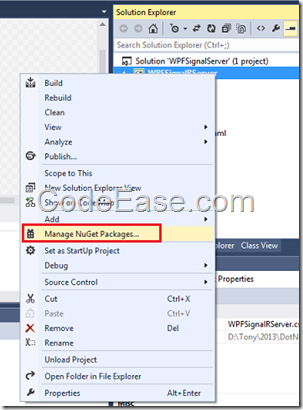
Install Microsoft ASP.NET SignalR Self Host package:
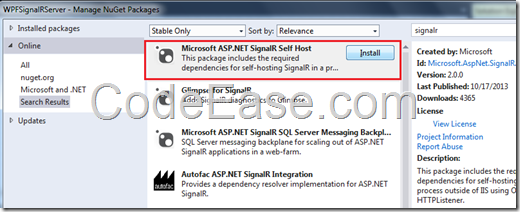
And Microsoft OWIN Cors:
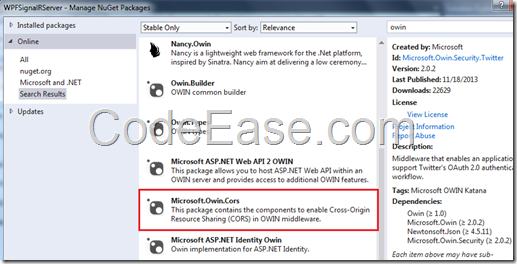
3: Now add a class file named Startup.cs, you can either add new class item or choose OWIN Startup class template to add the new item, but please remember to change the file name to Startup.cs
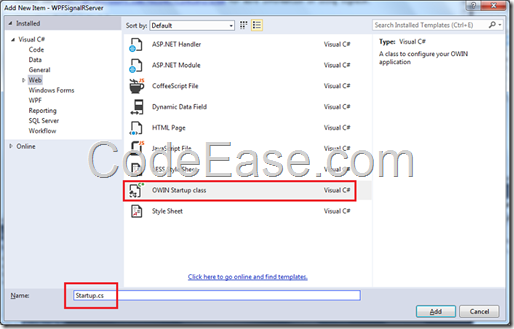
4: Now we just steal Patrick’s code to put in Startup Class:
using Microsoft.Owin;
using Owin;
using Microsoft.Owin.Cors;
public class Startup
{
public void Configuration(IAppBuilder app)
{
app.UseCors(CorsOptions.AllowAll);
app.MapSignalR();
}
}
5: Add a new Class file named MyHub.cs, and steal Patrick’s MyHub code:
public class MyHub : Hub
{
public void Send(string name, string message)
{
Clients.All.addMessage(name, message);
}
}
OK, you have seen my differences: put code into separate files
6: OK, there is really difference in this step! IF you continue to steal Patrick’s code, you will get trouble ( I did ).
Open MainWindows.xaml.cs file, add one line code:
IDisposable MySignalR { get; set; }
Then add Loaded event and copy the following code:
private void Window_Loaded(object sender, RoutedEventArgs e)
{
try
{
string url = "http://localhost:8374/";
this.MySignalR = WebApp.Start<Startup>(url);
if (this.MySignalR == null)
MessageBox.Show("web app started failed !");
else
MessageBox.Show("web app started! Try to visit " + url + "signalr/hubs");
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
Then Closing event code:
private void Window_Closing(object sender, System.ComponentModel.CancelEventArgs e)
{
if (this.MySignalR != null)
{
this.MySignalR.Dispose();
this.MySignalR = null;
}
}
Should add "using Microsoft.Owin.Hosting;" also.
7: Build the application and run it, you will see the following pop-up message:
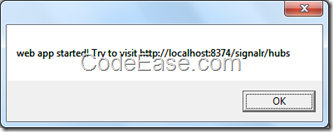
8: Now go to your web browser to visit the address: http://localhost:8374/signalr/hubs
(I used Chrome here. )
You should see the following information in Chrome web browser, otherwise it means you have made some mistakes in your code.
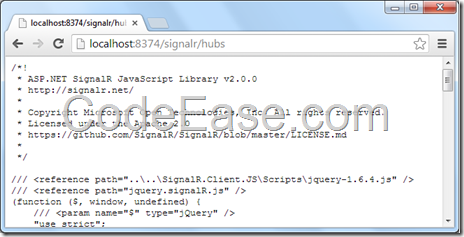
Now it indicates your SignalR 2 hosting WPF application successfully. You can continue to steal Patrick’s code to build a HTML+JavaScript client to try his chat application